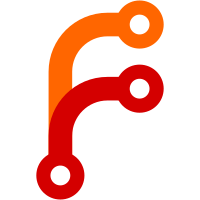
Hardcoded VAda Flickies Many thanks to MI for his help, even if he has sinful opinions on what the collection of creatures should be called. ;P * Flickies are now handled via A_FlickySpawn instead of hardcoded in P_KillMobj, so there can be mobjtypes with MF_ENEMY which don't create flickies, or other mechanisms which can much easier. * Added map header "FlickyList" (aka "AnimalList") parameter, which can either be set to: * A species (eg: "Rabbit" or "Bluebird", amongst 17 currently supported types in dehacked.c table FLICKYTYPES - including the seed from Sonic CD, which isn't limited to 'soniccd on' in the console now) * Any valid mobjtype that isn't MT_NULL (eg: "MT_FLICKY_GHOST") * A comma-seperated list of either of the above, up to 64 entries long (eg: "Cow,MT_FLICKY_SPIDER,Chicken") * "All" - sets behind-the-scenes stuff to use every 'normal' type of flicky in FLICKYTYPES (a distinction which can be utilised to hide secret level flickies where they wouldn't be appropriate for the main game) * "Demo" - sets behind-the-scenes stuff to use the five flickies closest to the species used in the game's long history. * "None" - prevents any flickies from spawning. "Demo" is functionally the default value if you don't include a FlickyList parameter in the header at all. Of note, a bunch of functions are now created: * A_FlickySpawn - spawns flicky. * A_FlickyAim - aims for area near target, but not directly on them - turns around when hitting wall * A_FlickyFly - flies/swims around target (calls A_FlickyAim) * A_FlickySoar - hacky alternate fly (calls A_FlickyAim) * A_FlickyCoast - slowing down before going off again * A_FlickyHop - fracunit-scale precision for A_BunnyHop * A_FlickyFlounder - A_FlickyHop with randomisation * A_FlickyCheck - State-setter for falling, or being on-ground * A_FlickyHeightCheck - State-setter for falling, or being below a certain height relative to target * A_FlickyFlutter - A_FlickyCheck, but with a slow fall/movement (calls A_FlickyCheck and A_FlickyAim) I don't need to enumerate the object types and states that have been added, do I? Oh yeah, I also made it so get_mobjtype's failure value was MT_NULL and prohibited SOC from editing the properties of it to compensate. IN ADDITION: Killed "soniccd" console command, since it made things more complicated and honestly being able to specify Sonic CD seeds in the level header is a better option. See merge request !60
83 lines
2.4 KiB
C
83 lines
2.4 KiB
C
// SONIC ROBO BLAST 2
|
|
//-----------------------------------------------------------------------------
|
|
// Copyright (C) 1993-1996 by id Software, Inc.
|
|
// Copyright (C) 1998-2000 by DooM Legacy Team.
|
|
// Copyright (C) 1999-2016 by Sonic Team Junior.
|
|
//
|
|
// This program is free software distributed under the
|
|
// terms of the GNU General Public License, version 2.
|
|
// See the 'LICENSE' file for more details.
|
|
//-----------------------------------------------------------------------------
|
|
/// \file p_setup.h
|
|
/// \brief Setup a game, startup stuff
|
|
|
|
#ifndef __P_SETUP__
|
|
#define __P_SETUP__
|
|
|
|
#include "doomdata.h"
|
|
#include "doomstat.h"
|
|
#include "r_defs.h"
|
|
|
|
// map md5, sent to players via PT_SERVERINFO
|
|
extern unsigned char mapmd5[16];
|
|
|
|
// Player spawn spots for deathmatch.
|
|
#define MAX_DM_STARTS 64
|
|
extern mapthing_t *deathmatchstarts[MAX_DM_STARTS];
|
|
extern INT32 numdmstarts, numcoopstarts, numredctfstarts, numbluectfstarts;
|
|
|
|
extern boolean levelloading;
|
|
|
|
extern lumpnum_t lastloadedmaplumpnum; // for comparative savegame
|
|
//
|
|
// MAP used flats lookup table
|
|
//
|
|
typedef struct
|
|
{
|
|
char name[9]; // resource name from wad
|
|
lumpnum_t lumpnum; // lump number of the flat
|
|
|
|
// for flat animation
|
|
lumpnum_t baselumpnum;
|
|
INT32 animseq; // start pos. in the anim sequence
|
|
INT32 numpics;
|
|
INT32 speed;
|
|
} levelflat_t;
|
|
|
|
extern size_t numlevelflats;
|
|
extern levelflat_t *levelflats;
|
|
INT32 P_AddLevelFlat(const char *flatname, levelflat_t *levelflat);
|
|
INT32 P_AddLevelFlatRuntime(const char *flatname);
|
|
INT32 P_CheckLevelFlat(const char *flatname);
|
|
|
|
extern size_t nummapthings;
|
|
extern mapthing_t *mapthings;
|
|
|
|
void P_SetupLevelSky(INT32 skynum, boolean global);
|
|
#ifdef SCANTHINGS
|
|
void P_ScanThings(INT16 mapnum, INT16 wadnum, INT16 lumpnum);
|
|
#endif
|
|
void P_LoadThingsOnly(void);
|
|
boolean P_SetupLevel(boolean skipprecip);
|
|
boolean P_AddWadFile(const char *wadfilename, char **firstmapname);
|
|
#ifdef DELFILE
|
|
boolean P_DelWadFile(void);
|
|
#endif
|
|
boolean P_RunSOC(const char *socfilename);
|
|
void P_WriteThings(lumpnum_t lump);
|
|
size_t P_PrecacheLevelFlats(void);
|
|
void P_AllocMapHeader(INT16 i);
|
|
|
|
void P_SetDemoFlickies(INT16 i);
|
|
void P_DeleteFlickies(INT16 i);
|
|
|
|
// Needed for NiGHTS
|
|
void P_ReloadRings(void);
|
|
void P_DeleteGrades(INT16 i);
|
|
void P_AddGradesForMare(INT16 i, UINT8 mare, char *gtext);
|
|
UINT8 P_GetGrade(UINT32 pscore, INT16 map, UINT8 mare);
|
|
UINT8 P_HasGrades(INT16 map, UINT8 mare);
|
|
UINT32 P_GetScoreForGrade(INT16 map, UINT8 mare, UINT8 grade);
|
|
|
|
#endif
|